How to Upload an Image in PHP and Store it in the Folder

In this tutorial, I will show you the exact steps on how to upload an image in PHP and store it in the folder.
Letās see how weāll do it:
First of all, weāll create a basic HTML form that will allow users to choose an image file from their pc and submit it to the form handler. A form handler is a file with a nameĀ upload.php
Ā that contains a PHP script.
This PHP script will upload the image to a folder that is located on a server. So, this is a straightforward process that weāre going to do now.
Our file structure will look like this.
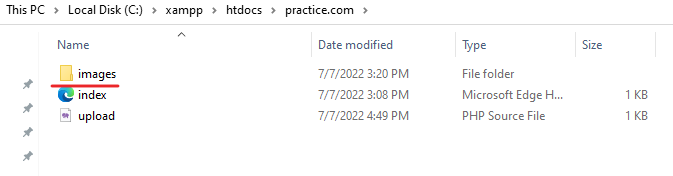
In this above file structure image, first I created my project folder inside āhtdocs
ā folder and named itĀ practice.com
. Then, I created 2 files and a folder inside it.
The images folder is where I will upload the image using PHP and store it in this folder. And theĀ index.html
Ā file will have HTML form code whileĀ upload.php
Ā file consists of a PHP script that will process images.
Now, just paste theĀ practice.com
Ā folder into your text editor, and letās code.
Tip:Ā If you donāt know to run PHP code on your pc then check this guide first onĀ how to run PHP codeĀ on your pc.
Letās begin with our first step:
Step 1: Create a Basic HTML Form to Upload Images in PHP
The form is very simple and it will look like this in your browser.
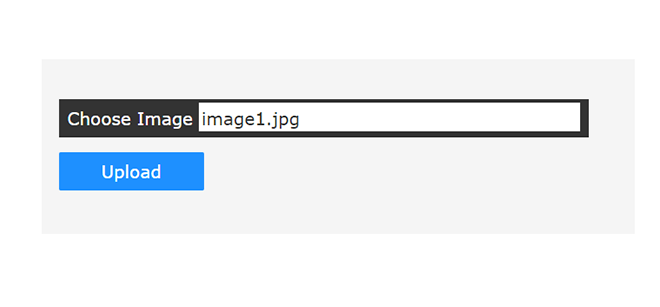
The form contains only one input to choose an image and an upload button. This form will allow users to choose an image to upload.
The HTML code of our image upload form will look like this below:
ā index.html
<form action="upload.php" method="POST" enctype="multipart/form-data"> <input type="file" name="imageToUpload"> <input type="submit" name="submit" value="Submit"> </form>
Code Explanation:
- In the above code, the form element contains three attributes, the firstĀ
action
Ā attribute is used for specifying the form handler file which isĀupload.php
. And the method we will use to send input to the upload.php file isĀPOST
. - And the third attribute I used isĀ
enctype
Ā the attribute and set its value to āmultipart/form-data
ā. Itās crucial if you want to upload an image file in PHP. - Inside the form element, I used two inputs, one is a file upload button and the second is a submit button which will upload images in a folder upon click.
And CSS code to style the form is below.
ā style.css
*{ box-sizing: border-box; margin: 0; font-family: Verdana, Geneva, Tahoma, sans-serif; font-size: 20px; } body{ padding-top: 100px; } form{ width: 50%; margin: auto; background-color: #f5f5f5; padding: 50px 20px; } input{ padding: 4px; } input[type=text]{ width: 100%; } input[type=file]{ background-color: #ffffff; color: #333; padding: 6px 3px 3px 3px; } input[type=file]::-webkit-file-upload-button { display: none; background-color: #333; } .file-upload{ background-color: #333333; color: #ffffff; padding: 10px; cursor: pointer; } input[type=submit]{ background-color: dodgerblue; color: #ffffff; padding: 10px; cursor: pointer; width: 26%; border-radius: 2px; border: none; } input[type=submit]:hover{ background-color: rgb(15, 116, 218); } @media screen and (max-width: 700px){ *{ font-size: 18px; } form{ width: 90%; } }
You can copy the above CSS code and paste it in a separate style sheet or inside style tags of HTML.
Now, our form is ready. Letās see the second step.
Step 2: PHP Code to Upload and Store Image in Folder
After choosing the image, when the user will click on the submit button. The HTML form will send data to theĀ upload.php
Ā file which weāre going to create now.
Inside theĀ upload.php
Ā file, weāll write some code to upload an image file and store it in a folder.
So, letās editĀ upload.php
Ā file in a text editor. Just see the code below.
ā upload.php
<?php if(isset($_FILES['imageToUpload'])){ move_uploaded_file($_FILES['imageToUpload']['tmp_name'], "images/". $_FILES['imageToUpload']['name']); } else{ echo "image not found!"; } ?>
Code Explanation:
1- First of all, on line number 2 in the above code, I checked whether theĀ name
Ā attribute of theĀ <input type="file">Ā
is set or not using theĀ if()
Ā statement withĀ isset()
Ā function.
Inside the function, I used theĀ $_FILES[ ]
Ā superglobal variable and pass the value of the name attribute which is āimageToUploadā.
If the variable is set and the statement will be true then the code inside it will execute otherwise it will not run.
2- Inside the curly brackets ofĀ if()
Ā statement, I usedĀ move_uploaded_file()
Ā function. This function is used to upload an image in PHP and store it in a folder.
TheĀ move_uploaded_file()
Ā function uses two parameters inside it.
ā php code
<?php move_uploaded_file(tmp_name, filepath.withName); ?>
The first parameter isĀ tmp_nameĀ which is the temporary name of the file.
And the second parameter is aĀ filename with the pathĀ where the image file will be stored.
These few lines of code will do the job and you can upload multiple images in PHP and store them in a folder.
This is how you can upload images in PHP and store them in a folder.
If you have questions related to this guide then feel free to leave a comment below.