How to Build a JavaScript Calculator (Code Included!)
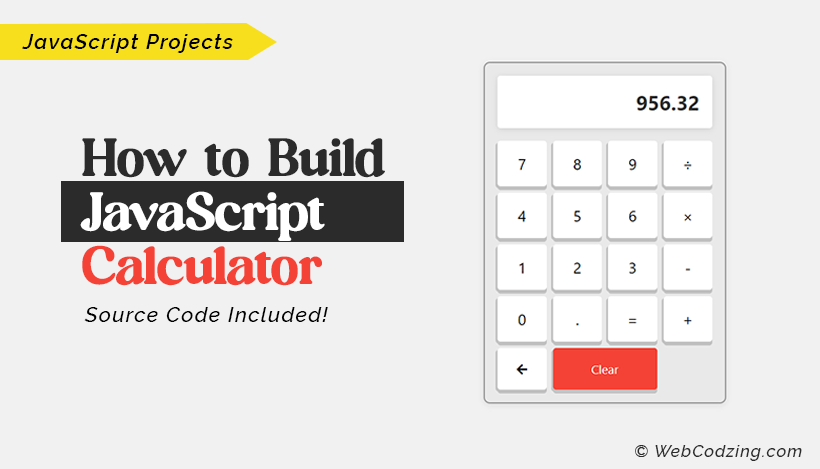
A JavaScript calculator web app is a digital tool that allows users to perform mathematical calculations directly in their web browser. It is one of the best web app ideas to work on. With this calculator web app, users can input numbers, perform arithmetic operations like addition, subtraction, multiplication, and division, and obtain accurate results instantly.
In this tutorial, we will use HTML, CSS, and JavaScript to walk you through the process of building a complete calculator from scratch. In the end, the JavaScript calculator will look like this as shown below.
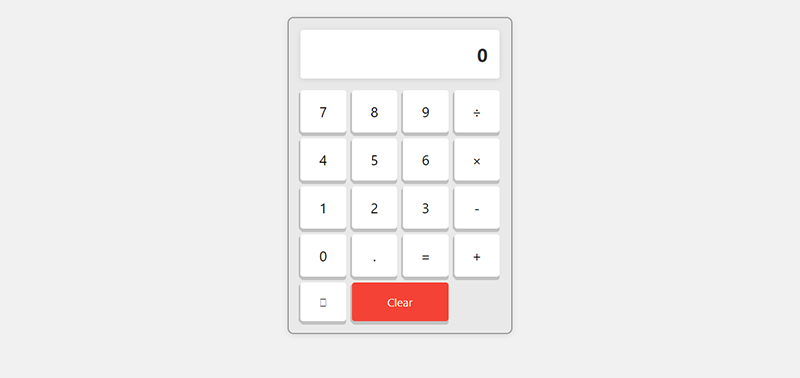
This tutorial will explain all the code used in making this calculator. And also, the code download link is included at the end of this tutorial.
Table Of Contents
It’s time to take a look at the steps to create a JavaScript calculator. So let’s get started!
Building JavaScript Calculator
This tutorial is divided into three main parts HTML, CSS, and JavaScript which show everything from scratch.
Before starting part 1, create a project folder on your PC and open that folder in your text editor as shown below.
Create Project Folder (Name it: JavaScript Calculator or whatever)

Open the project folder inside your text or code editor. (I’m using Visual Studio Code)
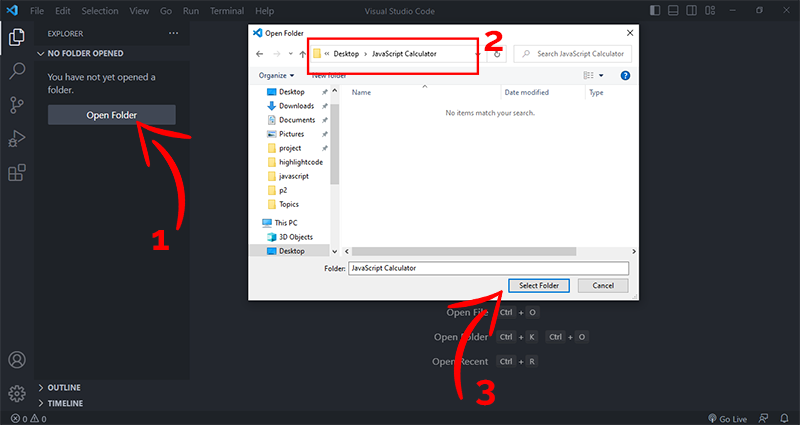
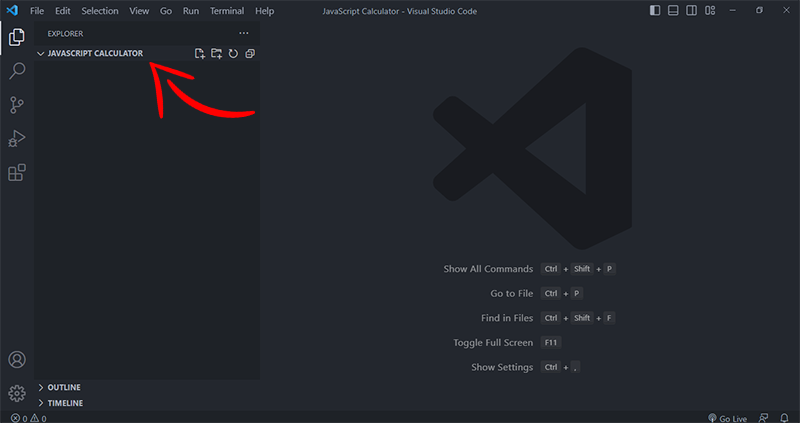
Now, let’s begin with the first part.
Part 1: HTML Structure of Calculator
First, we will use HTML to define the content of our calculator. Such as buttons for numbers, and arithmetic symbols for addition, subtraction, dividing, multiplying, etc.
Create a new HTML File
To do this, open your text editor and create a new HTML file in your text editor and name it index.html as shown below.

Now, the HTML file is added to your project folder. It’s time to write the code and build the basic structure of the calculator using HTML.
Build Calculator Layout
Look at the following, it’s the most basic HTML code that contains a pair of <html> tags, <head> section with <title> tags, and <body> tags in which we will code our calculator’s buttons and layout. All you have to do is just copy and paste the code. And then see the next step.
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Calculator</title>
</head>
<body>
<!-- The Calculator Code will go here -->
</body>
</html>
Next, define the calculator layout. Create three containers using <div> tags inside the <body> of HTML as shown below. In the below code, there is one parent container and two child containers in which one for displaying results and one will consist of the calculator buttons.
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Calculator</title>
</head>
<body>
<!--Calculator Container-->
<div class="calculator">
<!-- Calculator Result Screen -->
<div class="result" id="result">0</div>
<!-- Calculator Buttons Container-->
<div class="calculator-buttons">
<!-- Calculator Buttons will go here -->
</div>
</div>
</body>
</html>
To create some buttons, you will use <div> tags. In the following code, I created all the required buttons for our JavaScript calculator. Each button is defined using a <div> tag and has a class name buttons. You will use these classes to define CSS styles for each button of the calculator to make it beautiful. And every div element consists of a number.
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Calculator</title>
</head>
<body>
<!--Calculator Container-->
<div class="calculator">
<!-- Calculator Result Screen -->
<div class="result" id="result">0</div>
<!-- Calculator Buttons -->
<div class="calculator-buttons">
<div class="button">7</div>
<div class="button">8</div>
<div class="button">9</div>
<div class="button">÷</div>
<div class="button">4</div>
<div class="button">5</div>
<div class="button">6</div>
<div class="button">×</div>
<div class="button">1</div>
<div class="button">2</div>
<div class="button">3</div>
<div class="button">-</div>
<div class="button">0</div>
<div class="button">.</div>
<div class="button">=</div>
<div class="button">+</div>
<div class="button delete"></div>
<div class="button clear"></div>
</div>
</div>
</body>
</html>
Each line of the HTML code serves a specific purpose, such as defining the structure of the document and creating elements for the calculator layout.
If we open this HTML file in the browser then it will look something like this below.
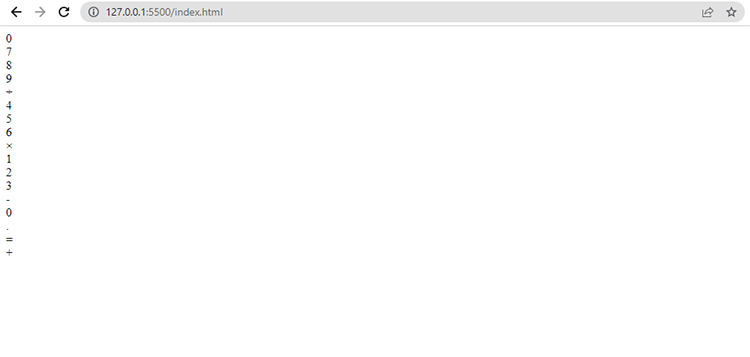
The basic HTML part of the JavaScript calculator is completed. Let’s see the CSS part to style our calculator using some CSS rules.
Part 2: Styling Calculator using CSS
We’ll use CSS in this calculator to define the visual appearance and layout of the HTML elements. By applying CSS styles, you can enhance the visual appeal of the calculator and make it more user-friendly.
Create and Link a CSS File to HTML
- To do this, first of all, create a separate CSS file in your text editor and name it style.css as shown in the image below.

2- Now, you need to link the CSS to the HTML file using the <link> tag because we’re using an external stylesheet. To do this, simply put the following code in the <head> section of your HTML.
<link rel="stylesheet" href="style.css">
The <head> section will look like this below.
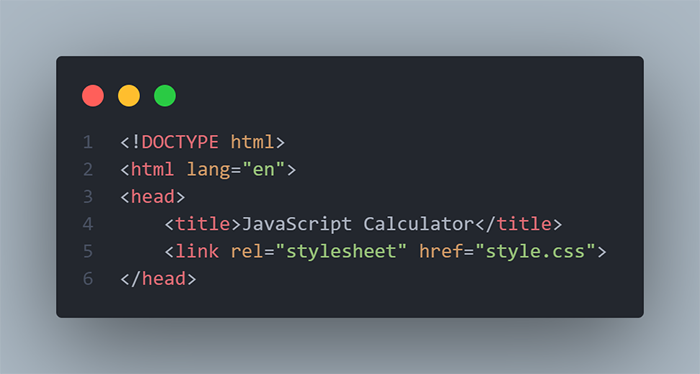
Now that CSS is linked to the HTML, let’s apply custom styling to the JavaScript Calculator.
Add Custom CSS Styling to Calculator
The following CSS rules will set the background color of the <body>
element to #f1f1f1
, which is a light gray color. It provides a background color for the entire page. And
body {
background-color: #f1f1f1;
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
}
.calculator {
max-width: 340px;
margin: 30px auto;
padding: 20px;
border: 2px solid #999999;
border-radius: 10px;
background-color: #e9e9e9;
box-shadow: 0px 2px 10px rgba(0, 0, 0, 0.1);
}
The output is shown below.
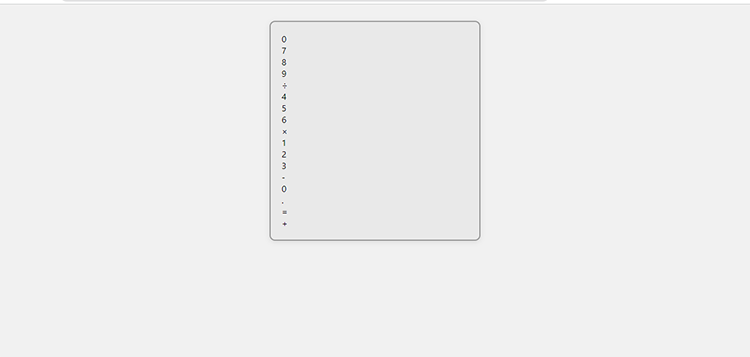
Add the following CSS rules to your stylesheet for buttons and the results section of the calculator.
.result {
text-align: right;
padding: 20px;
font-weight: bold;
color: #333333;
font-size: 2rem;
background-color: #ffffff;
border-radius: 5px;
box-shadow: 0px 2px 10px rgba(0, 0, 0, 0.1);
margin-bottom: 20px;
}
.calculator-buttons {
display: grid;
grid-template-columns: repeat(4, 1fr);
grid-gap: 10px;
}
.button {
padding: 20px;
text-align: center;
font-size: 1.5rem;
background-color: #ffffff;
border: none;
border-radius: 5px;
box-shadow: -3px 3px 0px #c2c2c2, 0px 6px 0px #c2c2c2, 0px 6px 0px #e9e9e9;
cursor: pointer;
transition: background-color 0.2s ease, transform 0.3s ease, box-shadow 0.3s ease;
}
.button:hover {
background-color: #f5f5f5;
transform: translateY(-2px);
box-shadow: -3px 3px 0px #c2c2c2, 0px 3px 0px #c2c2c2, 0px 6px 0px #e9e9e9;
}
.button:active {
background-color: #e1e1e1;
transform: translateY(2px);
box-shadow: -3px 3px 0px #c2c2c2, 0px 0px 0px #c2c2c2, 0px 0px 0px #e9e9e9;
}
.button:last-child {
grid-column: span 2;
}
.button.delete {
font-size: 1.2rem;
}
.button.delete:before {
content: "\f060";
font-family: "Font Awesome 5 Free";
font-weight: 900;
}
.button.delete:active {
background-color: #e9e9e9;
}
.button.clear {
font-size: 1.2rem;
background-color: #f44336;
color: #ffffff;
}
.button.clear:before {
content: "Clear";
}
.button.clear:active {
background-color: #d32f2f;
}
Output:
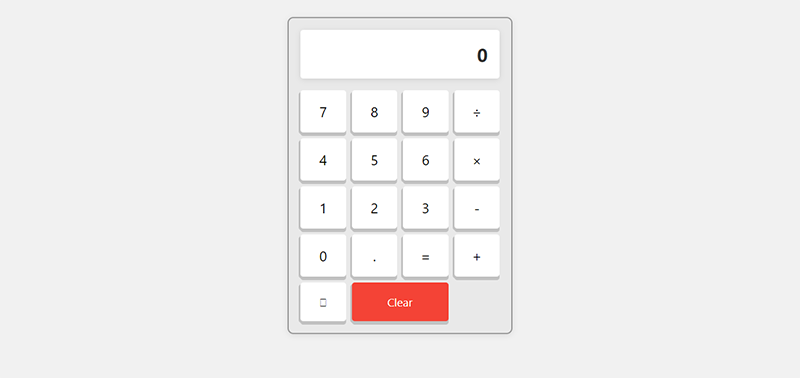
Part 3: Implementing Functionality to Calculator using JavaScript
JavaScript is the soul of
Create and Link a JavaScript File to HTML
1- First, create a separate JavaScript file and name it scripts.js as shown below.
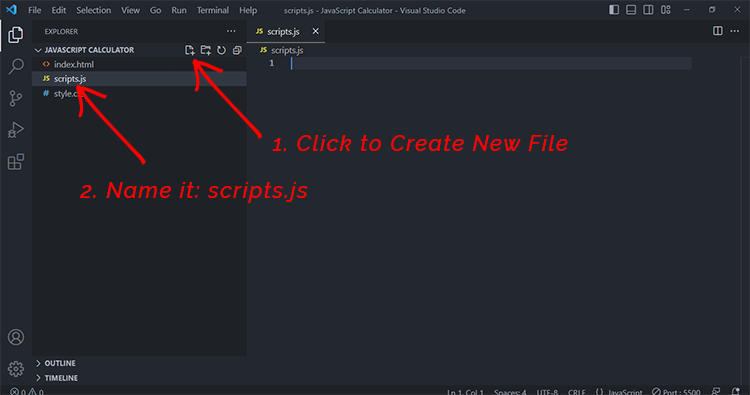
2- Link the JavaScript file to HMTL using the <script> tags as shown below.
<script src="scripts.js"></script>
Put the above <script> element in the <head> section of your HTML code to link JavaScript as shown below.

Define JavaScript Functions to Handle the calculator operations
We will create JavaScript functions to add different functionalities to our calculator.
1- appendToResult():
This function is called when a button is clicked and appends the clicked value to the result display. It takes the value
parameter, which represents the value of the clicked button.
The following JavaScript code shows the syntax to use this function.
function appendToResult(value) {
var result = document.getElementById('result');
if (result.textContent === '0') {
result.textContent = value;
} else {
result.textContent += value;
}
}
To make this function work, you need to add a click event to each button of the JavaScript Calculator. For this, use onclick attribute and update the HTML code as shown below.
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Calculator</title>
<link rel="stylesheet" href="style.css">
<script src="scripts.js"></script>
</head>
<body>
<!--Calculator Container-->
<div class="calculator">
<!-- Calculator Result Screen -->
<div class="result" id="result">0</div>
<!-- Calculator Buttons -->
<div class="calculator-buttons">
<div class="button" onclick="appendToResult('7')">7</div>
<div class="button" onclick="appendToResult('8')">8</div>
<div class="button" onclick="appendToResult('9')">9</div>
<div class="button" onclick="appendToResult('/')">÷</div>
<div class="button" onclick="appendToResult('4')">4</div>
<div class="button" onclick="appendToResult('5')">5</div>
<div class="button" onclick="appendToResult('6')">6</div>
<div class="button" onclick="appendToResult('*')">×</div>
<div class="button" onclick="appendToResult('1')">1</div>
<div class="button" onclick="appendToResult('2')">2</div>
<div class="button" onclick="appendToResult('3')">3</div>
<div class="button" onclick="appendToResult('-')">-</div>
<div class="button" onclick="appendToResult('0')">0</div>
<div class="button" onclick="appendToResult('.')">.</div>
<!--For Result-->
<div class="button" onclick="calculateResult()">=</div>
<div class="button" onclick="appendToResult('+')">+</div>
<!-- To Remove Last Charater-->
<div class="button delete" onclick="deleteLastCharacter()"></div>
<!-- To Clear Result -->
<div class="button clear" onclick="clearResult()"></div>
</div>
</div>
</body>
</html>
In the above HTML code, I update the buttons and added onclick=”appendToResult(value)”. Also, I used calculateResult() for the result, deleteLastCharacter() to remove the last character and clearResult() function to clear the screen. You will see the JavaScript code in a minute.
Now, what the appendToResult() function does is that it first retrieves the current result display by accessing the textContent
property of the result element. It then appends the clicked value to the end of the result display by concatenating the value with the current result. Finally, it updates the result display by assigning the modified result back to the textContent
property of the result element.
2- calculateResult() Function
This function is called when the equal (=) button is clicked to calculate and display the final result in JavaScript Calculator. It retrieves the current result display using the textContent
property of the result element.
The following code shows the syntax to use it.
function calculateResult() {
var result = document.getElementById('result');
var expression = result.textContent;
var answer = eval(expression);
result.textContent = answer;
}
The function uses the JavaScript eval()
function to evaluate the mathematical expression represented by the result display. The evaluated result is stored in a variable. Finally, the function updates the result display by assigning the calculated result to the textContent
property of the result element.
3- deleteLastCharacter() Function
This function is called when the delete (DEL) button is clicked to remove the last character from the result display. It first retrieves the current result display using the textContent
property of the result element.
Below is the syntax to use this function:
function deleteLastCharacter() {
var result = document.getElementById('result');
result.textContent = result.textContent.slice(0, -1);
}
The function uses the slice()
method to remove the last character from the result display by specifying the start and end index. The modified result is stored in a variable. Finally, the function updates the result display by assigning the modified result to the textContent
property of the result element.
4- clearResult() to Clear Result
This function is called when the clear button is clicked to clear the result display. It simply sets the textContent
property of the result element to an empty string, effectively clearing the result display.
function clearResult() {
var result = document.getElementById('result');
result.textContent = '0';
}
Lastly, I also added a font-awesome CDN link to the<head> section of HTML to use the icon for the last character remove button. The font-awesome link is shown below, add it inside the <head> section otherwise, the icon will not appear.
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.3/css/all.min.css">
That’s all. Hope this tutorial will be helpful.
Here’s the download link that contains the complete code of the JavaScript Calculator in a zip file.
If you have any questions related to this tutorial then feel free to ask in the comments section given below.
Don’t forget to help others by sharing this tutorial!