JavaScript getElementById() Method
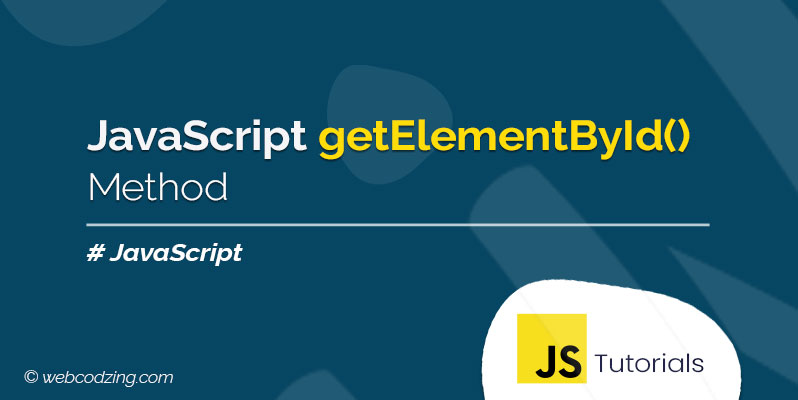
The JavaScript getElementById() is a method of DOM (Document Object Model). It is used to access or select HTML elements by their unique specified id’s name. So that we can remove, add, replace, or modify the content of the HTML elements.
The JavaScript code in the following example changes the color of the paragraph element with the assigned id=”demo”.
<h2>This is Heading</h2> <p>This is a paragraph 1</p> <p id="demo">This is a paragraph 2</p> <p>This is a paragraph 3</p> <!-- JavaScript Code --> <script> document.getElementById('demo').style.color = "blue"; </script>
Code Output:
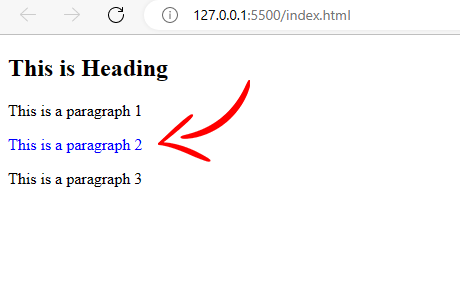
It does exactly what it sounds like, when we use this in JavaScript, the getElementById() method selects the HTML elements by their id.
As I said earlier the word “DOM”, if you want to know more about this then see this guide about Introduction to DOM.
How to Use the getElementById() Method properly
The following code shows the syntax to use getElementById() in JavaScript:
document.getElementById("id-name-here"); // write id name e.g., demo inside double quotes or single quotes
You must have to assign a unique name to the id attribute of the desired HTML element to use getElementById() method in JavaScript.
It works like a JavaScript function that takes only one argument in the form of an Id. In the above example, the argument is Id’s name, e.g., a demo inside parenthesis.
The function tells JavaScript the id of the HTML element that we want to access from the HTML document tree.
The important thing to remember here is, the JavaScript getElementById() method is case-sensitive. Mean if you write GetElementById() instead of getElementById() then it will not work. You have to use the exact syntax.
However, you can use single or double quotes to enclose the id’s name.
The following code will print “Hi, this is JavaScript” inside the second paragraph element with the id=”demo” using innerHTML.
<h2>This is Heading</h2> <p>This is a paragraph 1</p> <p id="demo">This is a paragraph 2</p> <p>This is a paragraph 3</p> <!-- JavaScript Code --> <script> document.getElementById('demo').innerHTML = "Hi, this is JavaScript!"; </script>
Code Output:

You can reduce the code by assigning it to a variable. The below JavaScript code creates a variable ‘x’ and assigns a document.getElementById(“demo”);
as its value.
<!-- JavaScript Code --> <script> let x = document.getElementById('demo'); </script>
Now, in this case, to write something inside innerHTML, you can write the code this way:
<!-- JavaScript Code --> <script> let x = document.getElementById('demo'); x.innerHTML = "Hi, this is JavaScript!"; </script>
The above code is short and we wrote “Hi, this is JavaScript!” inside HTML paragraph tags using the JavaScript document.getElementById() method.
Note: The id name must be unique and you can’t give one id name to more than one HTML element.
Hiding HTML Elements
You can hide the HTML element using document.getElementById().style.display as shown in the following code.
<h2>This is Heading</h2> <p>This is a paragraph 1</p> <p id="demo">This is a paragraph 2</p> <p>This is a paragraph 3</p> <!-- JavaScript Code --> <script> Document.getElementById('demo').style.display = "none"; </script>
Code Output:

Now, you know what JavaScript getElementById() method is and how to use it.
Also read: JavaScript getElementsByTagName()
Hope you did like this tutorial. If you have any questions related to this tutorial, you can ask them in the comment section below.