How to Check if Variable is a String in JavaScript

In JavaScript, we have several different methods to identify a string. This tutorial will teach you all the methods to check if a variable is a string in JavaScript. So, let’s see them one by one.
How to Check if a Variable is a String in JavaScript
Method 1: Use typeof Operator
The typeof
is the operator that allows us to determine the type of value that a variable holds. It returns the string that represents the data type of the operand.
The below syntax shows how to use the typeof
operator to check if a JavaScript variable is a string or not.
<script> // this is a variable named country var country = "United States"; // this will check if a variable is a string and return output document.write(typeof country); </script>
The code will output the data type on the screen as shown below.
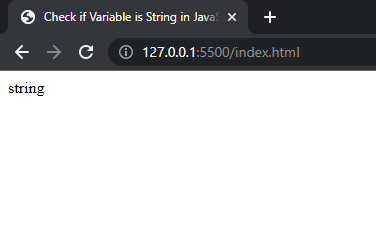
Let’s see another example which is a number instead of a string and let’s see what happens.
<script> // this is a variable named age var age = 36; // this code will check if a variable is a string or number and return output document.write(typeof age); </script>
Now, if you see the output below it shows the data type number means it’s working.
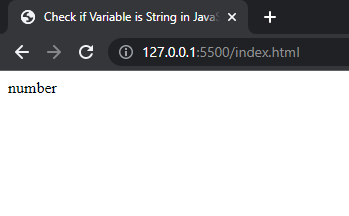
Code Explanation:
- In the above code, I just used two statements, first I created a variable with the keyword var and named it country or age, and provide the value.
- Then, I used a document.write() method with
typeof
the operator and variable name to check if the variable is a string in JavaScript.
Furthermore, you can use an if-else conditional statement to check if a variable is a string. The following code will do it.
<script> // this is a variable var myname = "zeeshan"; // this code will check if a variable is a string and return output if(typeof(myname) === 'string'){ document.write('The variable is a string'); } else { document.write('The variable is not a string'); } </script>
This first method is pretty simple, let’s see the second one.
Method 2: Use instanceof Operator
The instanceof
the operator is used to check if an object belongs to a specific class or constructor. And in JavaScript, strings are considered objects. So, this is also an effective way to check if a variable is a string in JavaScript.
The following code syntax is showing how to use instanceof
operator.
<script> // this is a variable var myname = new String('zeeshan'); // this code will check if a variable is a string and return output if(myname instanceof String){ document.write('The variable is a string'); } else { document.write('The variable is not a string'); } </script>
Output:
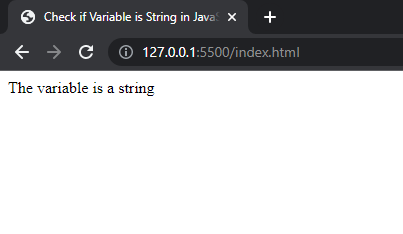
Code Explanation:
- First, I create a variable with the name ‘
myname
‘. I used the new string() method to create a variable. - Then, I used the if-else conditional statements to check if the variable is a string or not. In this statement, I used two strings that will show if the condition gets true or false.
That’s it. So this is how you can check if a variable is a string in JavaScript. If you have any questions then feel free to check in the comments section below.
Related Tutorial:
Plus, don’t forget to help others by sharing this tutorial!