Button with Rounded Corners using HTML CSS
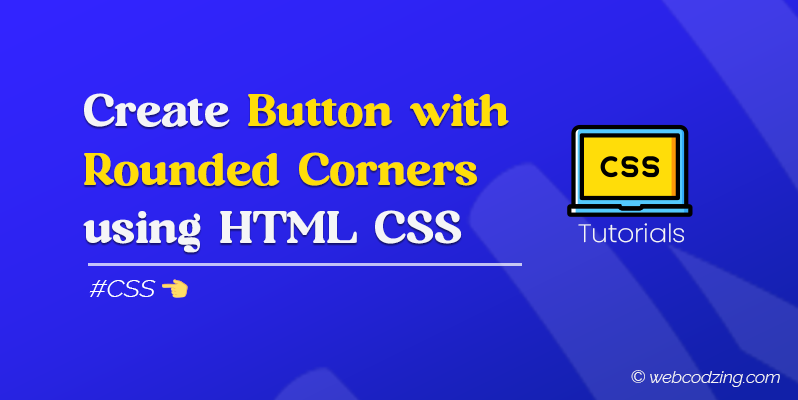
In this detailed tutorial, we will look at how to create a button with rounded corners using CSS step by step with code examples. Also, I will discuss different CSS properties border-radius
and more. Responsive design of the button and cross-browser compatibility are also discussed.
Rounded edges are one design feature that provides style and elegance to buttons. In web development, paying attention to design elements is crucial and significantly boosts the user experience.
Now, let’s jump in to see how to create a button with rounded corners with the help of HTML CSS.
Creating Buttons with Rounded Corners
To create a CSS button with rounded corners, we simply use the border-radius
property. The CSS border-radius
property controls the rounding of the corners of any HTML element whether it’s buttons, div containers, or images.
First of all, we need a simple HTML structure that includes the <button>
element we wish to modify. Here below is the HTML code for defining a button:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link rel="stylesheet" href="styles.css"> <title>Rounded Corner Button</title> </head> <body> <button class="rounded-button">Click me</button> </body> </html>
In this above code, I just used an HTML <button> element inside the body of HTML to create a button and give it a class named rounded-button.
Let’s now move on to the CSS part. First I will make a separate styles.css
file to keep our styles organized. Then, I will open styles.css
the file and add the following CSS styles to make the button look different.
.rounded-button { padding: 10px 20px; font-size: 16px; background-color: #3498db; /* Set your desired background color */ color: #ffffff; /* Set your desired text color */ border: none; cursor: pointer; } .rounded-button:hover { background-color: #217dbb; /* Set your desired hover background color */ }
The output of the above code will look like this below:
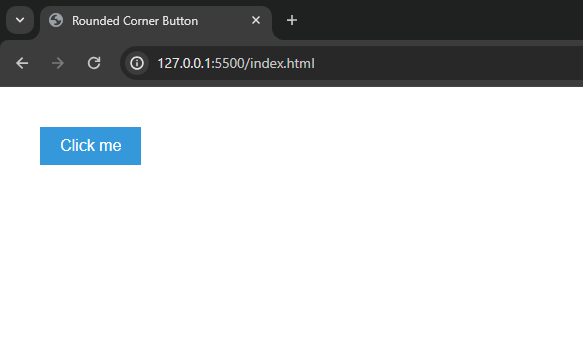
This code creates a simple button design with given padding, font size, colors, hover effects, etc.
Now, I will apply the border-radius
property to our button element which has the class=”rounded-button” as shown in the following CSS code. This will make the button with rounded corners.
.rounded-button{ border-radius: 10px; }
Now, if you see the code output below the button has rounded corners.
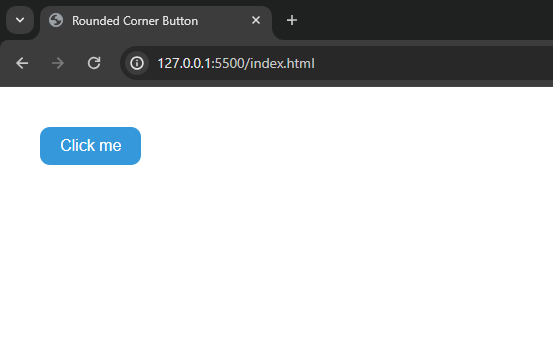
As you can see now, the button’s edges are quite round but if you want to make it look like a circle button then you can check out the tutorial about How to Make Circle Buttons with CSS.
CSS Code Explanation
- First of all, I used
.rounded-button
which is a CSS class selector to select an element by its class name. - Then, I used the
padding
property to set the inner spacing of a button around its text. - The
font-size
property will change the size of the text. - I used the blue background color of the button using the
background-color
property. - The
color
property will simply make the text white. - Then, the
border
property will remove the default border of the HTML button. - I used the cursor property to make the mouse look like a pointer when hovering over the button.
- Plus, I used
:hover
pseudo-class to change thebackground-color
of the button on hover. - Last of all, I applied
border-radius
property to make its corners rounded.
Now, let’s further talk about the border-radius property to make the custom corner of the button rounded. For example, as shown below in the image:
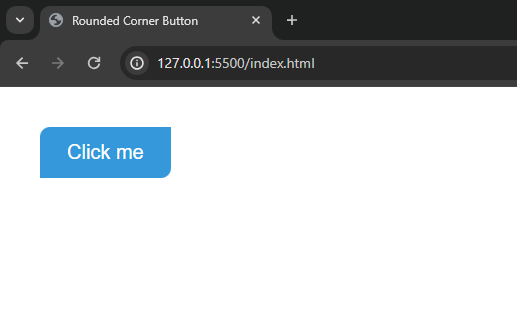
The border-radius Property
This property enables web developers to soften the edges of elements like buttons, divs, etc. To make a more eye-catching and usable design.
The border-radius property can have 4 different values which allows us to customize the custom corner of a button or container as shown below in the code example:
.rounded-button{ border-radius: 10px 0 10px 0; }
The above code will create a button which will look like this below:
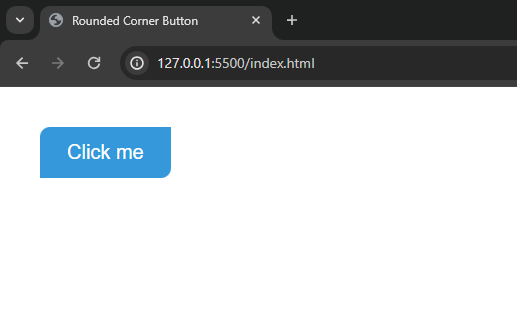
Furthermore, the 4 different values of this property work like this below.
border-radius: topleft topright bottomright bottomleft;
It works in a clockwise. The 1st value sets the top left corner radius, the 2nd value sets the top right corner border radius, the 3rd value sets the bottom right and the 4th value sets the bottom left corner radius.
In the above, I used a short-hand border-radius
property. You can also use a single property for each corner like this below:
.rounded-button{ border-top-left-radius: 10px; border-top-right-radius: 0; border-bottom-right-radius: 10px; border-bottom-left-radius: 0; }
Moreover, you can also specify the values in percentages instead of pixels.
Developers can adjust the curvature of the corners by setting the border-radius attribute to a certain value, such as 10px or 20px. This simple change may significantly improve the overall appearance and feel of buttons on a website.
Let’s talk about hover and transitions to make the animated button with rounded corners as shown below:
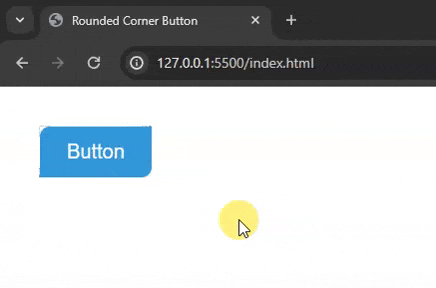
Applying Hover and Transitions to Rounded Button
The :hover
pseudo-class defines styles that will be applied when the mouse hovers over the button. Transitions are used to animate the button. Now, what I will do is I will use a combination of hover and transition properties to animate the corners on hover.
In the following CSS code, I applied the transition effect to border-radius
and changed it on hover.
.rounded-button { padding: 14px 27px; font-size: 20px; background-color: #3498db; color: #ffffff; border: none; cursor: pointer; border-radius: 10px 0 10px 0; transition: border-radius 0.3s ease; /* Adding a transition effect for the border-radius property */ } .rounded-button:hover { background-color: #217dbb; border-radius: 0px 10px 0px 10px; /* Changing the border-radius on hover */ }
Code Output:
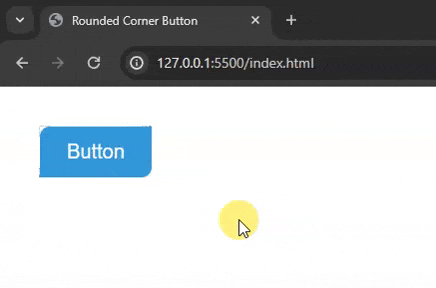
Tip: You can also create a button with a link! For that, check out this helpful tutorial about How to Create an HTML Button with a Link (4 Methods)
Advanced CSS Styling Techniques for Rounded Button
While the basic style principles remain unchanged, you may elevate your button design by using extra CSS elements. Here are some advanced strategies for improving your rounded button:
- Gradient Backgrounds: You can use gradient backgrounds to create depth and dimension in your button design. Here’s an example.
.rounded-button { background: linear-gradient(to right, #3498db, #2980b9); }
Change the gradient colors to match your project’s color scheme.
- Box shadow: Use a modest box shadow to add depth to your button. This creates the appearance of the button hovering just over the page.
.rounded-button { box-shadow: 0 4px 6px rgba(0, 0, 0, 0.1); }
Customize the shadow settings based on your design choices.
3. Icon Integration: Use icons in your buttons to convey extra information or actions. Use icon libraries or custom SVG icons and arrange them appropriately.
/* Icon Integration */ .rounded-button { padding-left: 30px; /* Adjust as needed */ background-image: url('icon.svg'); background-repeat: no-repeat; background-position: 10px center; /* Adjust the position */ }
Making Buttons Responsive
It’s important to make sure that your button with rounded corners appears fantastic on a variety of screens. Use responsive design concepts by using relative units for padding, font sizes, and border-radius.
For that purpose, consider using CSS media queries to customize button styles based on the device’s screen width.
In the following, I defined different media queries for both mobile and tablet devices so that the button with rounded corners works best on both devices.
/* Media query for tablet device */ @media screen and (max-width: 892px) { .rounded-button { padding: 14px 27px; font-size: 20px; background-color: #2980b9; } } /* Media query for mobile phones */ @media screen and (max-width: 580px) { .rounded-button { font-size: 16px; padding: 10px 20px; background-color: #3498db; } }
Furthermore, the transition effect must work on all browsers, for that we have to make the code compatible with other browsers.
Cross Browser Compatibility
Improving user interaction goes hand in hand with designing attractive buttons. Ensure that your buttons are accessible and work in all browsers, including those that have compatibility issues.
The following code will ensure that your code works on different browsers like Mozilla Firefox, Bing, Opera, etc. However, this will apply only if you’re animating your rounded buttons with transition.
/* Vendor prefixes for transition property */ .rounded-button, .rounded-button:hover { -webkit-transition: border-radius 0.3s ease, background-color 0.3s ease; -moz-transition: border-radius 0.3s ease, background-color 0.3s ease; -o-transition: border-radius 0.3s ease, background-color 0.3s ease; transition: border-radius 0.3s ease, background-color 0.3s ease; }
So, this is how you can create an HTML button with rounded corners with CSS. You can also copy and paste the above-provided code to use it in your web projects.
Conclusion
In this tutorial, you learned about creating buttons with rounded edges, their different styling techniques, responsiveness, cross-browser compatibility, and more. By combining CSS with advanced style choices, taking responsiveness into account, and focusing on user interaction, we develop buttons with rounded edges that not only improve the appearance of your website but also contribute to a smooth and delightful user experience.
Also, read about How to Create Transparent Buttons with CSS.
Keep experimenting with multiple styles, test your rounded button designs on different devices, and always aim for a balance of beauty and functionality in your web development projects.
Hope you did like this informative tutorial!
Don’t forget to help others by sharing this tutorial with others!