How to Hide Scrollbar CSS (3 Methods)

In this tutorial, you will learn 3 different methods on how to hide the scrollbar using CSS. So, let’s begin.
How to Hide / Remove Scrollbar with CSS
Method 1: Use CSS overflow Property
CSS gives us overflow
property to work with scrollbars. The overflow property has the following built-in values:
overflow: auto | hidden | scroll | visible;
We just need the overflow: hidden; property.
The overflow
is the shorthand property for overflow-x
and overflow-y
. The overflow-x
is for the horizontal scrollbar while overflow-y
is for the vertical scrollbar.
Let’s see how to use them with a code example.
Example 1.1: In the following, I used some HTML code to create a div container and added a paragraph with random text inside it.
<div class="container"> <p> Lorem ipsum dolor sit amet consectetur, adipisicing elit. Deleniti dignissimos, dolore, natus quia explicabo veritatis architecto praesentium eum voluptatem quasi cum tempora. Libero eveniet distinctio, ipsa dolores recusandae expedita. Nulla! Lorem ipsum dolor sit amet consectetur, adipisicing elit. Deleniti dignissimos, dolore, natus quia explicabo veritatis architecto praesentium eum voluptatem quasi cum tempora. Libero eveniet distinctio, ipsa dolores recusandae expedita. Nulla! </p> </div>
Then, I gave it a width
of 250px and a height
of 200px, and a border
and some padding
using the following CSS code.
div.container{ width: 280px; height: 200px; border: 2px solid black; padding: 10px; }
Code Output:
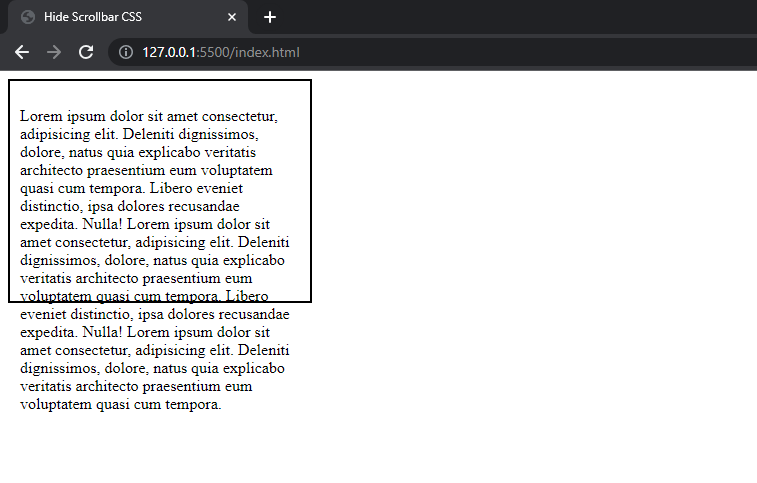
So what is happening in the above code output is that the text is flowing outside the container because the container is smaller. Here comes the overflow: auto;
property.
div.container{ width: 280px; height: 200px; border: 2px solid black; padding: 10px; /* Here I specified overflow */ overflow: auto; }
In the above, I used the overflow: auto;
property to adjust the text. The text is inside the container and the overflowing text is hidden but the vertical scrollbar is displayed as shown in the output below.
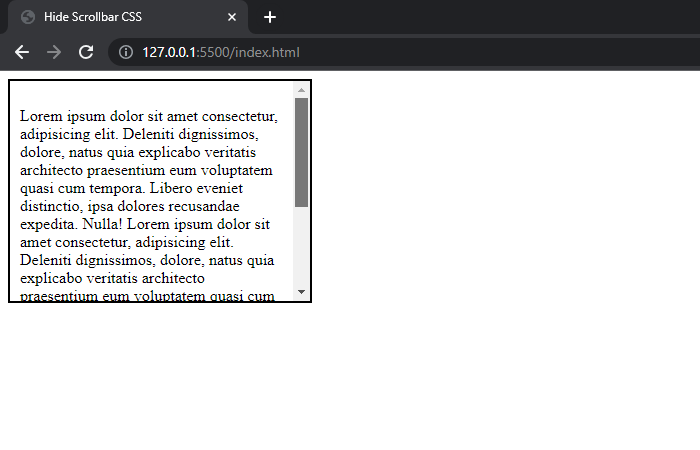
The scrollbar allows us to scroll to see the hidden text, but as the purpose of this tutorial is to hide scroll with CSS, let’s do it.
In this case, I will use overflow: hidden;
which will hide the scrollbar and in the meantime, it will also hide the text flowing outside the box.
div.container{ width: 280px; height: 200px; border: 2px solid black; padding: 10px; /* Here I specified overflow */ overflow: hidden; }
You can see in the below output the scrollbar has been removed. But…

But the problem is that the text is hidden and I can’t scroll to see the text. So, I want to hide the scrollbar with CSS but still scroll. So, this is the downside of using overflow: hidden;
property. But fortunately, we have another method to do that. So, let me introduce you to the second method.
Method 2: Use ::-webkit-scrollbar to Hide Scrollbar
The ::-webkit-scrollbar
is a CSS pseudo-element that is mainly used for customizing the scrollbars. The important thing is that it hides the scrollbar but you can still scroll. Let’s see how to use it.
The CSS syntax to hide the scrollbar but still scroll:
div.container::-webkit-scrollbar{ display: none; } div.container{ width: 280px; height: 200px; border: 2px solid black; padding: 10px; /* must specify overflow: auto; */ overflow: auto; }
In the above code, I used the div.container
with ::-webkit-scrollbar
and set the display
property none
to hide the scrollbar of the container. I used it with the combination of overflow: auto;. The rest of the code remains the same.
Note: The overflow: auto;
must be specified because it is necessary to make the content or text of the container scrollable.
The below output shows a text container with a removed scrollbar but you can still scroll down using your mouse wheel to see the hidden text.

Furthermore, if you use ::-webkit-scrollbar
without specifying a custom element as shown in the code below. Then it will hide all the scrollbars present on your screen even the browser’s main scrollbar on the left.
::-webkit-scrollbar{ display: none; }
Note: This code only works for Webkit-based browsers like Chrome, Safari, and Opera.
If you want to hide the scrollbars in the Firefox web browser then the next method is for you.
Method 3: Use scrollbar-width (for Firefox Browser only)
If you want to hide the scrollbar in the Firefox browser then the CSS scrollbar-width
property is the alternative of ::-webkit-scrollbar
. This property is introduced by Firefox and it works only for Firefox. All you have to do is, just setting it to none
.
The following code shows the syntax:
div.container{ scrollbar-width: none; }
So, the above two methods will hide the scrollbars but keep the functionality of scrolling.
Best Practices for Hiding Scrollbars
When hiding scrollbars you should consider the following best practices to ensure a better user experience:
- Ensure Accessibility: Always consider accessibility when you hide the scrollbar. Because different devices have different features and many users rely on scrollbars. For those users, it is crucial to make the content accessible through other means like a mouse wheel, and keyboard navigation buttons when you hide the scrollbar. For that, I recommend the second method.
- Don’t Disable Scrolling: When you use overflow: hidden; then it usually disables scrolling too. So, avoid using it.
- Always Consider Cross-Browser Compatibility: Test and run your code on different browsers for a better user experience all over the web.
That’s all.
So now you know how to remove or hide the scrollbar with CSS.
If you’re interested in learning about the overflow property in detail then check this tutorial:
Plus, Don’t forget to help others by sharing this tutorial!