JavaScript Get Viewport Width and Height

Are you finding a way to get viewport width and height in JavaScript? You can achieve this with just a few lines of JavaScript code. This tutorial will show you step by step process with examples.
Let’s see how to do this.
How to Get Viewport Width and Height using JavaScript
To get the current viewport width in JavaScript, we use the window object with .innerWidth and .innerHeight properties. The following code shows how to get viewport width and height in JavaScript.
<script>
// storing width and height values in variables
var width = window.innerWidth;
var height = window.innerHeight;
// code to display viewport width and height on screen
document.write(width + " x " + height);
</script>
The code will show the current width and height of your browser window. The output on my laptop is shown below, however, your browser window size might be different in that case you will see different values.
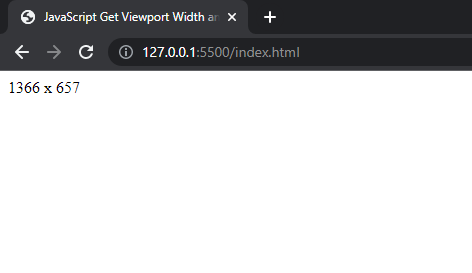
Note: The width and height values are in pixels.
Moreover, the above code will show the fixed viewport width and height of the current screen or window. But what if you resize your browser window? Then, the values remain the same. But you might expect the change. Here we recommend another appropriate way that will dynamically update the values of width and height if you resize your browser screen.
To get the viewport width and height dynamically in JavaScript, we use an event listener. The following code shows how to do it.
<script>
// creating a function to handle viewport change
function viewportHandler(){
document.write(window.innerWidth + " x " + window.innerHeight);
}
// Adding eventlistner for window resize
window.addEventListener("resize", viewportHandler);
// Calling viewportHandler() function when resize occurs
viewportHandler();
</script>
Code Explanation:
- First, I created a function that will be called when the resize event occurs.
- Then, I added the event listener for window resizing.
- And the last line of code will make a function call when the event will occur.
Note: After resizing your browser window, you have to refresh the web page to see the output.
Benefits of Getting Viewport Width in JavaScript
The major benefits of getting the viewport width and height in JavaScript are the following:
- It empowers front-end developers to create responsive web designs by checking the width and height of different screen sizes of any device.
- You can precisely position elements.
- Utilize media queries effectively, and enhance user interaction.
- By accessing the viewport width and height, you can adapt your website layout and content dynamically, ensuring optimal presentation across different devices and screen resolutions.
That’s all. This is how to get viewport width in JavaScript. If you have questions feel free to ask in the comments sections below.
Also read, how to disable right-clicking on the website using JavaScript.
Help others by sharing this tutorial!