JavaScript Hello World Program with Code Examples

This tutorial will teach you how to create a simple JavaScript âHello Worldâ Program. Youâll see four different ways to print âHello Worldâ in JavaScript with code examples and explanations.
âHello Worldâ is a traditional word and it is not mandatory you can use any other word. The basic purpose of creating the âHello Worldâ program is to illustrate to beginners how a JavaScript program works.
Before starting, if you donât know where to add JavaScript code in HTML. Then, you can check out our complete guide on how to add JavaScript to HTML.
Letâs begin.
How to Print Hello World in JavaScript
In JavaScript, we have four different ways to print âHello World!â.
innerHTML
 propertyâ to write inside an HTML elementalert()
 methodâ to create an alert boxdocument.write()
 methodâ use to output in HTML. Just for testing purposes, not recommendedconsole.log()
 â for debugging
Letâs see each method syntax to write Hello World using JavaScript.
1. Use innerHTML Property
This innerHTML method is used to print inside HTML elements. By using this method, you can print Hello World inside the HTML elements. We use it with the getElementsById() method.
Just see lines no. 12 to 14 in the code example given below. You can also copy the JavaScript Hello World script and paste it into your HTML document to see the result by yourself.
<!DOCTYPE html> <html lang="en-US"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=, initial-scale=1.0"> <title>A Simple Js Program</title> </head> <body> <h2 id="demo"></h2> <script> document.getElementById('demo').innerHTML = "Hello World!"; </script> </body> </html>
Code Explanation:
In the above code, I print âHello Worldâ in the h2 element. To do this first I specified the id attribute for the h2 element so that I can access this element through the document.getElementById method. The âHello Worldâ is enclosed in pair of double quotes because it is a text string. And it is the visible part that you will see in your browser.
However, you can use both double or single quotes. Inside the parenthesis or round brackets, I used the name of the id which is âdemoâ to write a text string inside the h2 element.
After the execution of the code, the output will look similar to the one below.
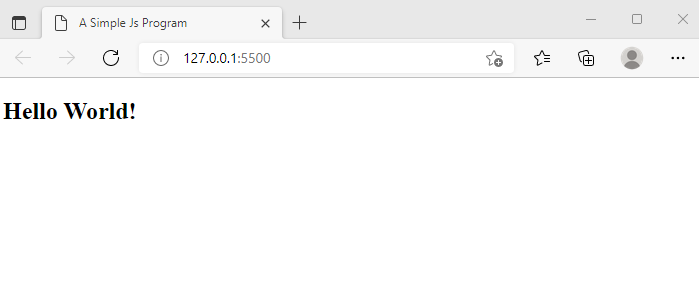
Tip: You can also access elements by JavaScript getElementsByTagName() method.
Letâs see the second method.
2. Use alert() Method to Print Hello World
This method is used to create an alert box in JavaScript. This alert box will appear when we open our HTML webpage or click a button. This is on us how we want JavaScript to show the alert box.
Letâs create a JavaScript Hello World alert box. The syntax to create the âHello Worldâ alert box is given below.
<!DOCTYPE html> <html lang="en-US"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=, initial-scale=1.0"> <title>A Simple Js Program</title> </head> <body> <script> alert("Hello World!"); </script> </body> </html>
Code Explanation: In the code above, I used an alert statement which is alert();. And inside the round brackets, I wrote the text string along with double quotes. And the JavaScript code is enclosed in the pair of <script> tags. If youâre using an external JavaScript file then <script>
 tags are not necessary.
You can copy the code and paste it at the end of <body> tags. Or you can paste it into your external JavaScript file without <script> tags. After saving the code, the output will be like this below.
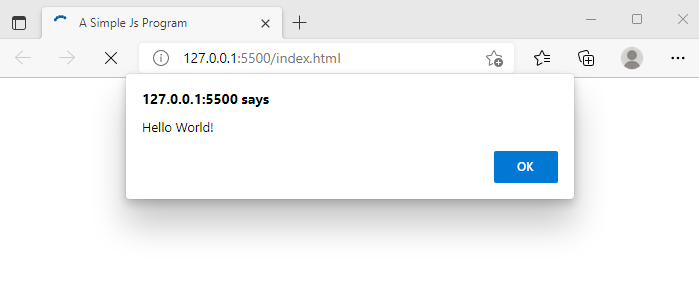
Letâs see the third method to output âHello World!â in JavaScript.
3. Use the document.write() Method
This method is for testing purposes we donât use it regularly and it is not recommended. The document.write() method directly prints in the body of HTML. Just see lines no. 11 to 13 in the code example below.
<!DOCTYPE html> <html lang="en-US"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=, initial-scale=1.0"> <title>A Simple Js Program</title> </head> <body> <script> document.write("Hello World!"); </script> </body> </html>
Code Explanation:
In the above code, I used a JavaScript document. write(); statement. And inside round brackets, I wrote text string. The output will look like this below.
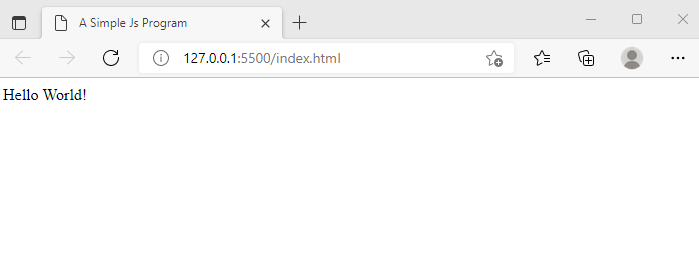
The downside of using this statement is that it overwrites HTML content. For example, you created a button through JavaScript and it will show a text line on click. But when you click the button all the previous HTML content is removed.
Letâs see the last method.
4. Use console.log() Method
We use console.log() for debugging purposes. In this method, we use our web browserâs console to write JavaScript code. The benefit of using the console.log is that it outputs the result on the same screen on the next line.
It really helps in debugging. You can write JavaScript code without any text editor using just your browser. Letâs print Hello World to the console.
Firstly, you need to open your browserâs console. To do this, open a new tab in your browser. Then, right-click and click inspect as shown below.
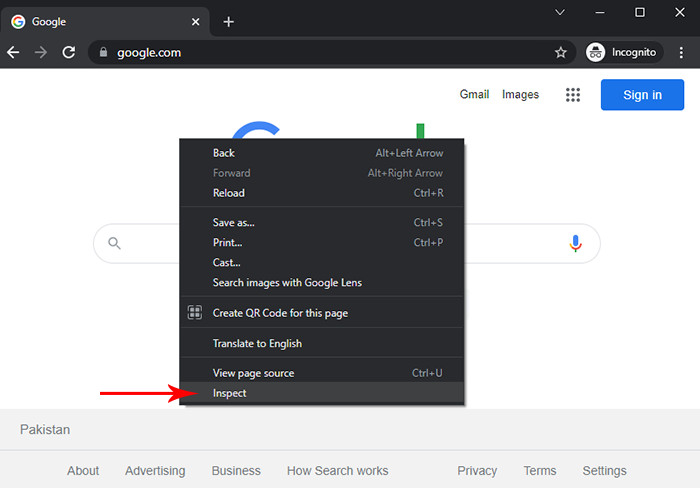
After that click on the console, and write your code there as shown below.

To print JavaScript âHello Worldâ in the console, just see the code example below.
console.log("Hello World!");
You can copy this line of code and paste it into your browserâs console. Then, click enter and you will see the result is the same as shown below.
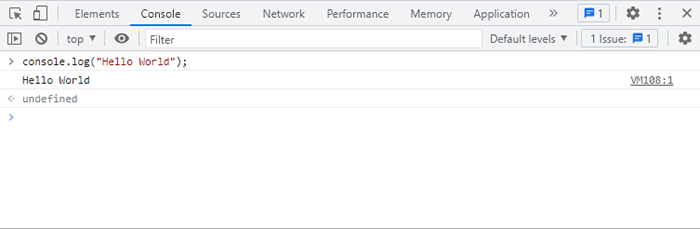
So, this is how you can create a simple JavaScript Hello World program.
Thatâs it. Hope you liked it. If you have any questions related to this tutorial then feel free to ask in the comments section below.
Donât forget to help others by sharing this tutorial!