6 Methods to Print Array in JavaScript
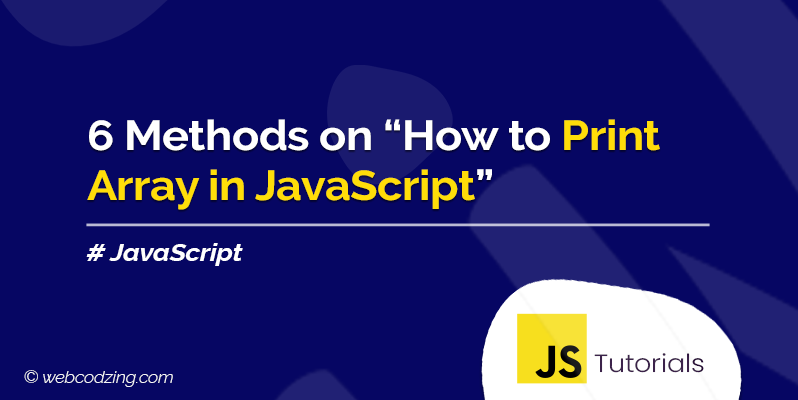
In this guide, we’ll explore various strategies to print an array in JavaScript with code examples. So, let’s begin without wasting any time.
JavaScript is the web language that gives developers vast tools for working with data. The array is one of its key data structures and is particularly useful for storing and organizing items. Before going to the solutions, let’s briefly introduce the JavaScript array.
What is a JavaScript Array?
An array is a type of object that allows you to store numerous values in the same variable. Each item in an array is referred to as an element, and they can be of any data type, such as integers, strings, objects, or other arrays.
For example, in the following, I have an array of numbers named myArray declared with let
keyword.
let myArray = [1, 2, 3, 4, 5];
Or you can see the following code which includes an array of multiple strings declared with var
keyword.
var myArray = [ "john", "nick", "tom", "zack" ];
Wondering, how to print this array. Let’s see all the methods.
How to Print Array in JavaScript
Method 1: Use console.log() to Print Array
The simplest way to print an array in JavaScript is to use the console.log()
function. This function is commonly used for both debugging and general output. When you send an array to console.log()
, the array’s contents are printed to the console.
In the following example, I created an array of numbers and then used a console.log()
function to print that array in the console.
// creating an array
let myArray = [1, 2, 3, 4, 5];
// printing through console.log() method
console.log(myArray);
The output of this code will be displayed in your browser’s console as shown below in the image:
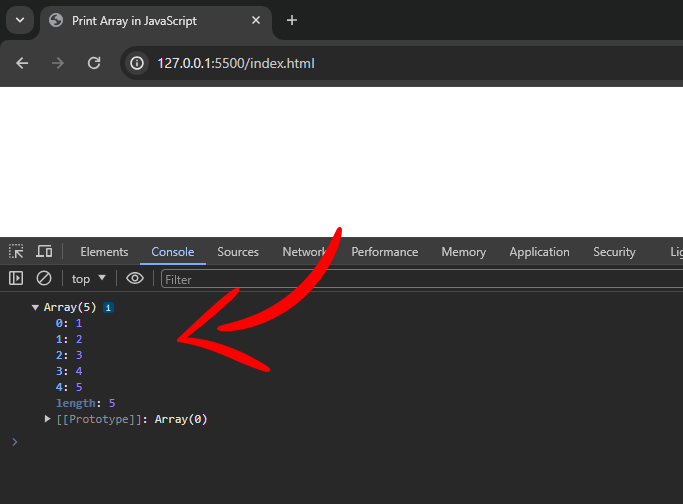
Furthermore, while dealing with online applications, you may wish to see array contents in the user interface on screen rather than the console. For that, let’s have a look at the second method.
Method 2: Use the document.write() method
The document.write()
method is a straightforward way to print array content on a web page, but it has significant limitations. Let’s see how to use it with a code example.
// Sample array
var myArray = [1, 2, 3, 4, 5];
// Using document.write() method to print the array
document.write("<p>Array printed using document.write: " + myArray + "</p>");
Let’s break the code:
- First, I created an array of numbers using the
var
keyword and named it myArray. - Then, I used a
document.write()
method with the array name inside it, to print that array in an HTML document. - I combined the array content with a string and wrapped it in paragraph (<p>) tags.
Code Output:
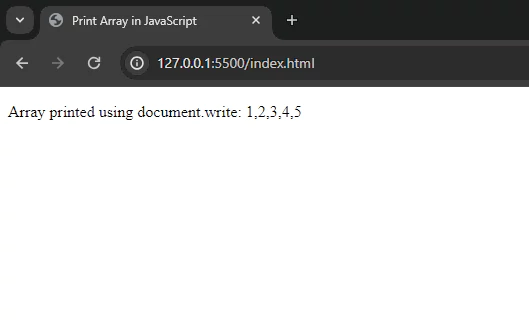
The document.write()
method overwrites the entire document if invoked after loading. It can have unexpected outcomes and is typically avoided in favor of modifying the DOM in more controlled ways. Due to this, document.writer()
method is rarely used in current web development.
However, we have loops 🙂 to print arrays. Let’s see that in the next method.
Method 3: Print Array in JavaScript using For Loop
You can utilize loops to cycle over an array and print each element individually to get more control and flexibility. Generally, using the for loop is the best way to do this.
In the following example, I used an array of strings named myArray. Then, I print that array using the for-loop syntax.
// Sample array
var myArray = [
"john",
"nick",
"tom",
"zack"
];
for (var i = 0; i < myArray.length; i++) {
document.write(myArray[i] + "<br>");
}
The output of this JavaScript code will look like this below:
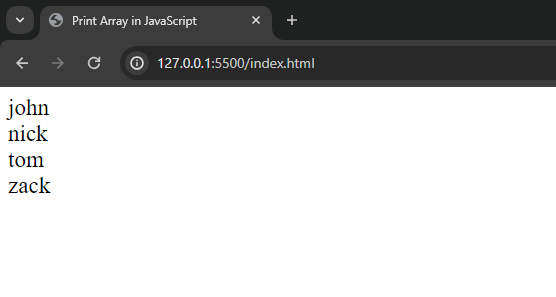
Loops are very beneficial whenever you have hundreds of items in an array. The ‘for loop‘ allows you to do extra actions within the loop body, making it ideal for more complicated applications.
Also, you can learn more about loops in this article about What is Loop in JavaScript?
Now, let’s go ahead to the next method.
Method 4: Use JavaScript alert() Method to Print Array
The JavaScript alert()
method is a basic approach for printing array elements in a pop-up window. It’s straightforward to use. Let’s look at the code example of how to use this alert() method to print an array in JavaScript.
// Sample array var myArray = [1, 2, 3, 4, 5]; // Using alert() to display the array alert("Array printed using alert:" + myArray);
Let’s break the code:
- First, I created an array of numbers.
- Then, I used the
alert()
method with the array name with a concatenated string inside it to print.
This method will invoke a pop-up dialog with the concatenated string containing the array content as shown in the code output below.
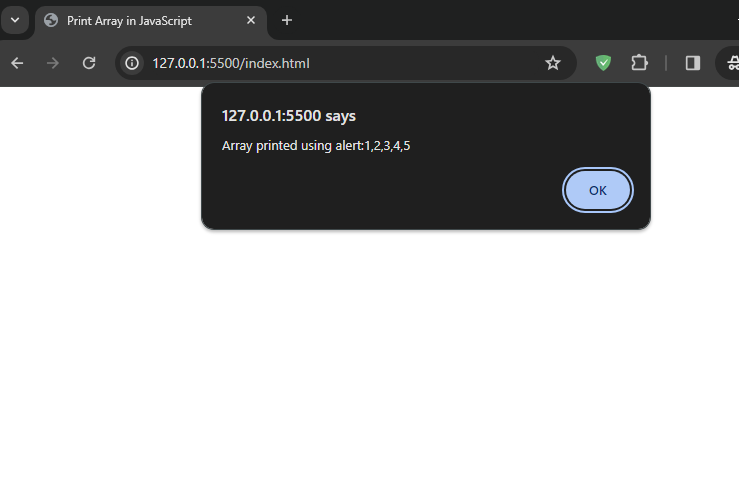
Note: Although alert()
is a straightforward method but sometimes it might disturb the user experience if not utilized appropriately.
Method 5: Display Arrays in HTML Elements
In web development, you frequently need to display array data on the user interface. Using HTML and JavaScript combined, you may dynamically display arrays on a webpage inside.
// creating array
var myArray = [1, 2, 3, 4, 5];
//creating div element
var outputContainer = document.createElement('div');
//using forEach to print array contents in HTML <p> elements
myArray.forEach(element => {
let elementNode = document.createElement('p');
elementNode.textContent = element;
outputContainer.appendChild(elementNode);
});
// printing on screen
document.body.appendChild(outputContainer);
The above code will create a <div>
container and print array items inside <p>
tags using JavaScript.
Code Explanation:
- First of all, I created an array of numbers as usual.
- Then, I used
forEach()
method with JavaScriptappendChild()
method. - This code will build HTML tags using the JavaScript document.createElement() method that generates p elements for each array element and attaches them to a div container. The container is later added to the body of the HTML text.
The Code Output will look like this below:
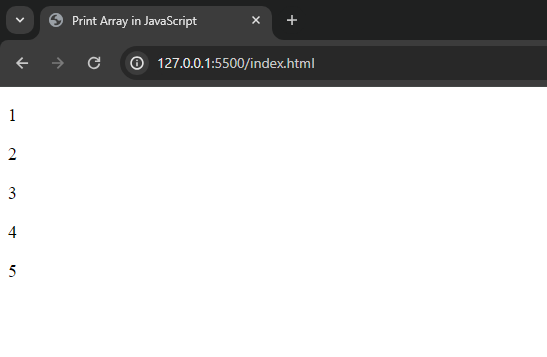
Method 6: Use forEach() method to Print Array
The forEach()
method is another technique, which is intended for arrays. It’s very simple and easy to use. Just have a look at the code example given below.
// creating an array
let myArray = [1, 2, 3, 4, 5];
// using forEach() method
myArray.forEach(element => {
document.write(element);
});
Code Output:
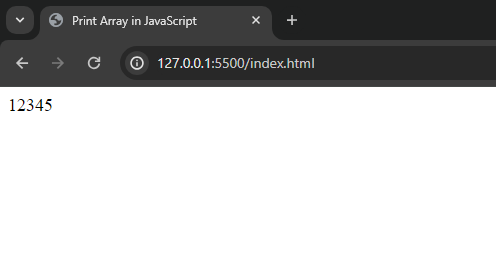
The forEach()
method simplifies the syntax and makes it easier to repeat across array elements.
You might think, What if I have a multi-dimensional array? So, let’s handle that problem.
How to Print Multi-Dimensional Arrays
When we work with multidimensional arrays meaning “arrays within arrays“, printing gets more complicated. You may use nest loops to browse both the outer and inner arrays. How?
Let’s see that in the following code example to print a multi-dimensional array in JavaScript.
let matrix = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ]; for (let i = 0; i < matrix.length; i++) { for (let j = 0; j < matrix[i].length; j++) { console.log(matrix[i][j]); } }
The above code contains a multi-dimensional array. The nested for loop
structure enables us to print elements from each subarray of the main array.
Code Output:

Conclusion
In this article, we saw 6 different methods to print arrays in JavaScript. Printing arrays in JavaScript requires simple techniques or methods, and each method is tailored to a distinct application. Understanding these techniques, whether you’re debugging, formatting output, or presenting data on a webpage, will allow you to work with arrays professionally.
So, this is how you can print arrays in JavaScript.
I hope you learned a lot from this guide.
Have fun learning JavaScript by building a simple calculator discussed in this guide about How to Build a JavaScript Calculator.
Just feel free to ask questions and also share this article with friends to help others.