CSS Forms
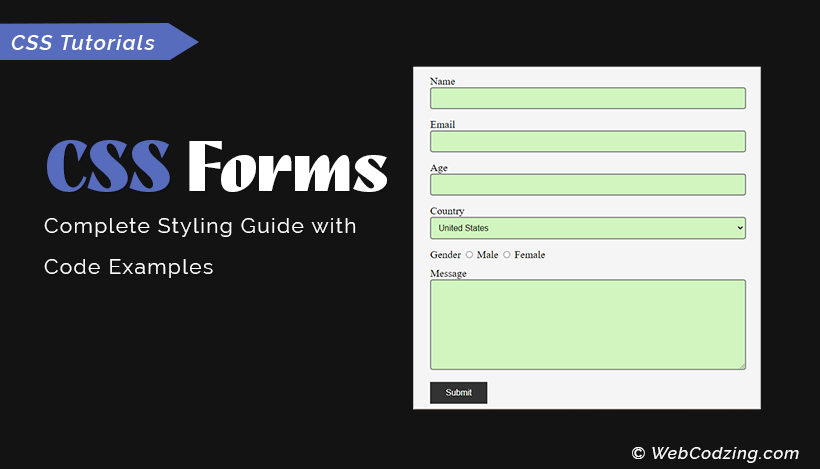
With CSS, you can improve the look and feel of HTML forms. You can make your HTML forms responsive and elegant. In this tutorial, we’ll explore different CSS properties that can be used to design HTML forms.
The CSS form we will design will look like this at the end.

Here below is the basic HTML form structure that we’re going to style with CSS. The complete HTML code for the form is given below.
<form action="" method="post"> <label for="name">Name</label><br> <input type="text"><br> <label for="email">Email</label><br> <input type="email"><br> <label for="age">Age</label><br> <input type="number"><br> <label for="country">Country</label><br> <select id="country" name="country"> <option value="United States">United States</option> <option value="India">India</option> <option value="uae">United Arab Emirates</option> <option value="Canada">Canada</option> </select><br> <label for="gender">Gender</label> <input type="radio"> Male <input type="radio"> Female <br> <label for="message">Message</label><br> <textarea name="msg" id="message" cols="30" rows="10"></textarea><br> <input type="submit"> </form>
The above form has 2 text input fields, 1 email field, 1 age field, a country selection dropdown, radio buttons for gender, and a text area for writing the message with a form submit button at the end. If you don’t know about the elements and tags that are used to create HTML forms then first you should check out this tutorial about HTML form elements complete guide.
However, the above HTML form code output will look like as shown below.
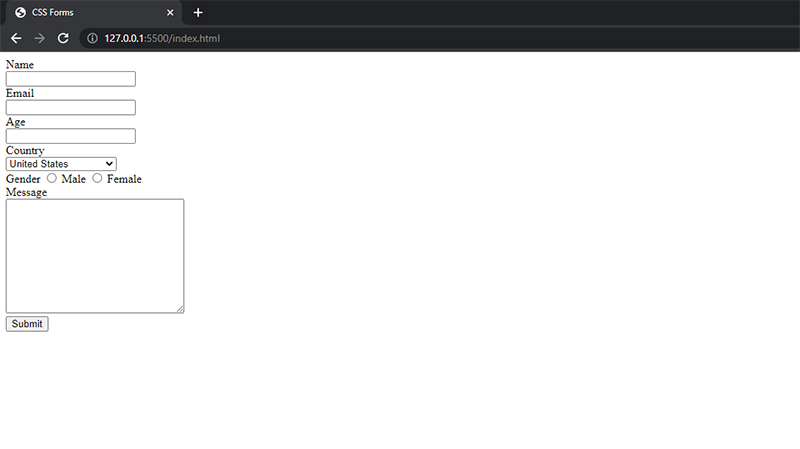
The form has not any CSS styling that’s why it is looking very simple and this is what we are going to discuss now.
With CSS, we can make our HTML form elegant and responsive which will look pretty and will work on any device screen. So, let’s begin with that.
CSS Form Width and Margin
First and foremost, we should define the form width so that we can place the form in the center of the screen. For this, I will apply the width property and set the width to 50% of the screen. And also will set the margin property to auto as shown in the CSS code below.
<style> form{ width: 50%; margin: auto; } </style>
Now, as you can see below our CSS form is in the center of the screen.

Tip: There are 2 more ways for centering a form that you can check in this tutorial about How to center a form in CSS.
CSS Form Background Color and Padding
Now, I will use the CSS background-color property to give our form a background color of gray. Which will make our form container with a width of 50% visible on the screen. And 50px padding to the <form> element.
<style> form{ width: 50%; margin: auto; background-color: #f5f5f5; padding: 50px; } </style>
Code output:

CSS Form Font Size
You can also modify the font size of your CSS form elements.
To make the font look better, bigger, and clear, I will apply the font-size property here as shown below.
<style> form{ width: 50%; margin: auto; background-color: #f5f5f5; padding: 50px; font-size: 20px; } </style>
Styling CSS Form Inputs
Inputs are the important elements of any HTML form we use them to gather input data from the user. We use CSS properties to make form inputs more beautiful and responsive on screen. Let’s see how each property can be used.
CSS Form Inputs Padding
Input padding is the inner spacing of an input field. The syntax to adjust the padding of form inputs is given below.
<style> input, select, textarea{ padding: 10px; } </style>
In this CSS code above, I used element selects with comma separation. Which are input, select, and textarea. These will access the HTML elements and apply the styles to them.
Now, if you see the below output the input fields are bigger than before.
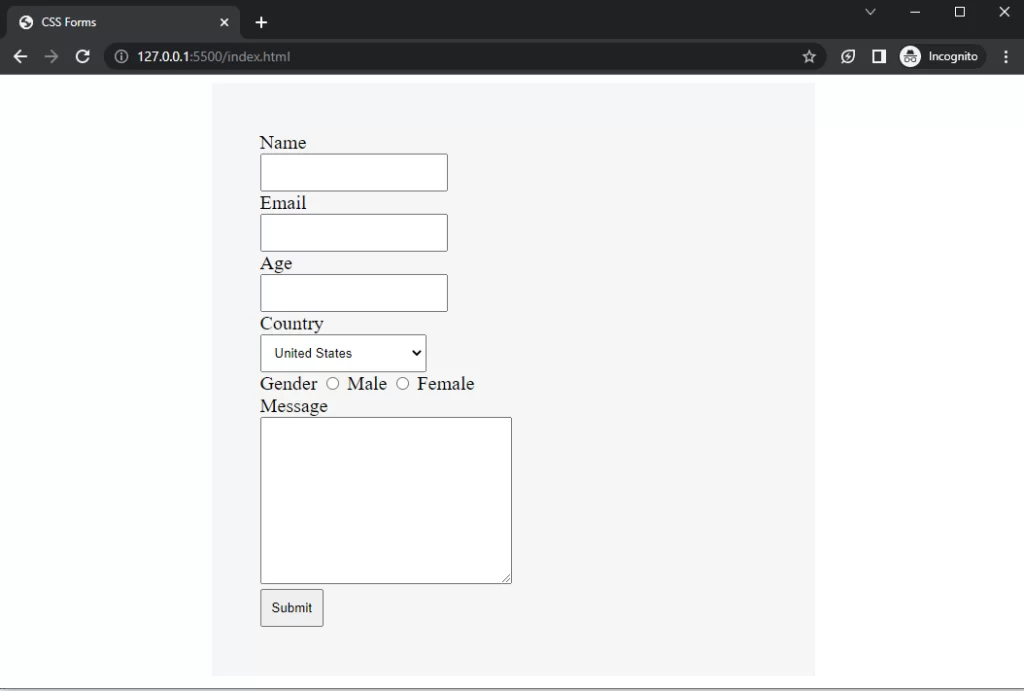
Also, later in this tutorial, we’ll see how to adjust the spacing outside input fields.
CSS Form Inputs Width
To make your form inputs look wider, you can use the width property and set its width to anything like 100% for full-width form input fields.
<style> *{ box-sizing: border-box; } form{ width: 50%; margin: auto; background-color: #f5f5f5; padding: 30px; font-size: 20px; } input[type=text], input[type=email], input[type=number], select, textarea{ padding: 10px; width: 100%; } input, select, textarea, label{ margin-bottom: 10px; } </style>
In the above code, I used attribute selectors to select different input fields for example,
- input[type=text]Â will select all text input fields.
- input[type=email]Â will select email input fields
- input[type=number]Â will select the number of input fields
Code Output:
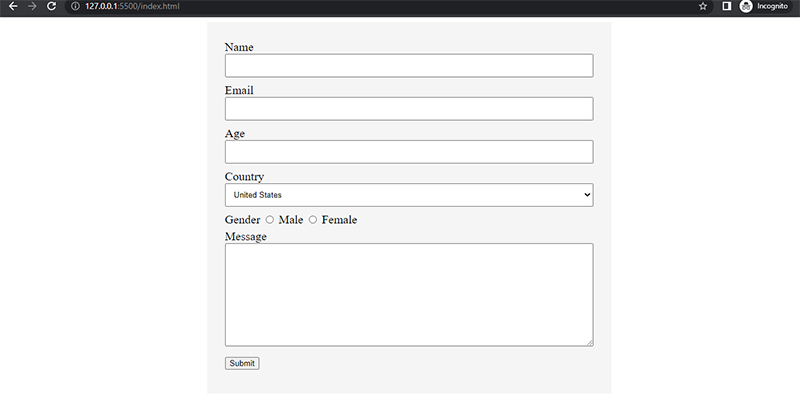
Margin around CSS Form Inputs
We use the CSS margin property to adjust the spacing around the form input elements.
I will use the margin-bottom property to set the bottom margin for every input field.
input, select, textarea{ margin-bottom: 18px; }
Form Button Styling using CSS
We can use padding, background color, and border properties to change button styling.
The following CSS code will make the button wider and will change its background color.
input[type=submit]{ padding: 12px 30px; border: none; background-color: #333333; color: #ffffff; font-size: 16px; }
Code Output:

Form Input Borders
You can set borders for input fields using the CSS border property as shown in the code below.
input[type=text], input[type=email], input[type=number], select, textarea{ border: 2px solid gray; }
Code Output

However, you can also use border-right, border-left, border-top, and border-bottom properties to set the border of one or more sides.
You can also use the border-radius property to set the radius of an input. Just set the value to any number, the syntax is shown below.
input[type=text], input[type=email], input[type=number], select, textarea{ border: 2px solid gray; border-radius: 5px; }
Now, the border-radius is shown below at each input field corner.

Form Inputs Background Color
The input fields’ background color can be set using the background-color property for CSS form.
The code below will set the color for text input fields, email input, age, dropdown, and textarea.
input[type=text], input[type=email], input[type=number], select, textarea{ background-color: #D0F5BE; }
Output is shown below:

Focused Inputs
Focused inputs mean whenever you will click on an input field it will trigger CSS styles. For example, you can change the background color or border when click on the input field.
To create a focused input, we use the :focus
 selector.
The CSS code below will change the background color of all input fields from green to white when clicked:
input:focus{ background-color: #ffffff; }
Output:

However, the same process applies if you want to change the border color of input fields when focusing on CSS forms.
Complete CSS Code for Form Styling
<style> *{ box-sizing: border-box; } input, select{ font-size: 16px; } form{ width: 50%; margin: auto; background-color: #f5f5f5; padding: 30px; font-size: 20px; } input[type=text], input[type=email], input[type=number], select, textarea{ padding: 10px; width: 100%; border: 2px solid gray; border-radius: 5px; background-color: #D0F5BE; } input, select, textarea, label{ margin-bottom: 10px; } input[type=submit]{ padding: 12px 30px; border: none; background-color: #333333; color: #ffffff; font-size: 16px; } input, select, textarea{ margin-bottom: 18px; } </style>
The box-sizing property at the top of the CSS code is used to add padding and borders of an element in the width and height of the element.
That’s all. This is all about CSS forms.
Now, you know how to style HTML forms with CSS. If you have any questions related to this tutorial then ask in the comment section.