How to Center a Form in CSS (3 Methods)
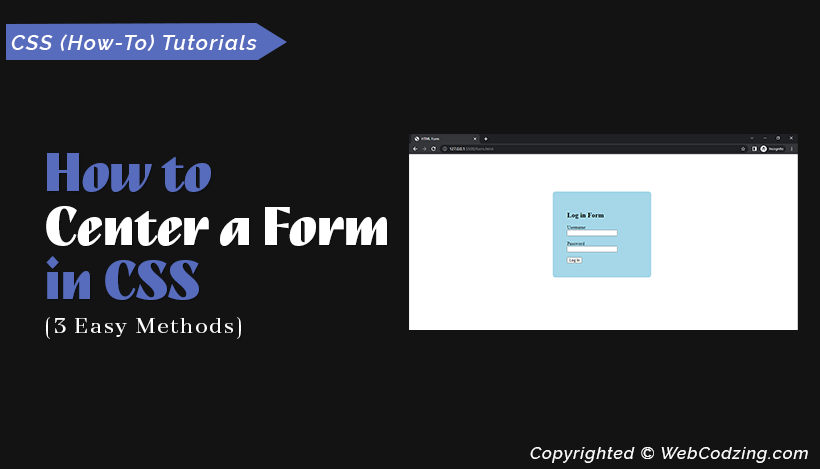
We have three different ways to center a form in CSS horizontally. Centering a form is an easy task that you can achieve with just a few lines of code. This tutorial will show you how to do this step by step.
The HTML form that we will use in this guide is shown below. It’s simple but will do the job.
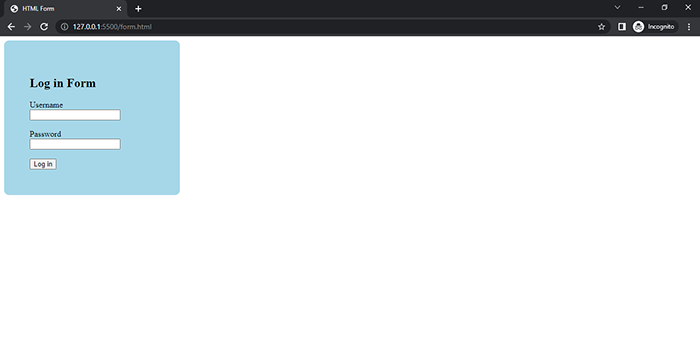
And the HTML code of the above form that we will edit is given below.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>HTML Form</title> <style> form{ width: 18%; padding: 50px; border-radius: 10px; background-color: #A5D7E8; } </style> </head> <body> <div class="form"> <form action=""> <h2>Log in Form</h2> <label for="uname">Username</label><br> <input type="text"><br><br> <label for="password">Password</label><br> <input type="password"><br><br> <input type="submit" value="Log in"> </form> </div> </body> </html>
Remember in the upcoming examples we will make changes to the above HTML form code.
Also, if you don’t know how to create an HTML form then check out this complete tutorial about how to create a contact form in HTML.
Let’s learn how to center a form horizontally in CSS.
Center a Form in CSS
Method 1: Use CSS margin property
The very simplest and easiest method to center a form horizontally in CSS is by using the margin property. How? Let’s see.
First, you need to set the width for the form element and it’s crucial. And background color (optional) so the form container can be visible. The CSS code is shown below.
form{ width: 18%; background-color: #A5D7E8; padding: 50px; border-radius: 10px; }
I also added some padding and border-radius too. But you can do it according to your choice.
Now, simply set the CSS margin to auto, and your HTML form is centered.
form{ width: 18%; background-color: #A5D7E8; padding: 50px; border-radius: 10px; margin: auto; }
If you see the login form in your browser, it is aligned horizontally as shown in the image below.
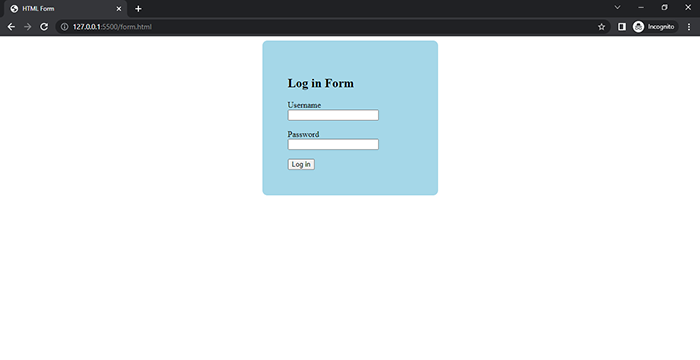
You can check out more about CSS margin property here. Let’s see the second method.
Method 2: Use CSS Flexbox to Center Form
CSS flexbox is another best way to center an HTML form in CSS. All you have to do is just enclose the form in <div> tags to make a container using CSS. This way we can set the flexbox property in CSS. The HTML code is below.
<div class="container"> <form action=""> <h2>Log in Form</h2> <label for="uname">Username</label><br> <input type="text"><br><br> <label for="password">Password</label><br> <input type="password"><br><br> <input type="submit" value="Log in"> </form> </div>
Now, go to the CSS code and set the display property to flex for the div. container element as shown below.
<style> div.container{ display: flex; justify-content: center; } form{ width: 18%; padding: 50px; border-radius: 10px; background-color: #A5D7E8; } </style>
Code Output:
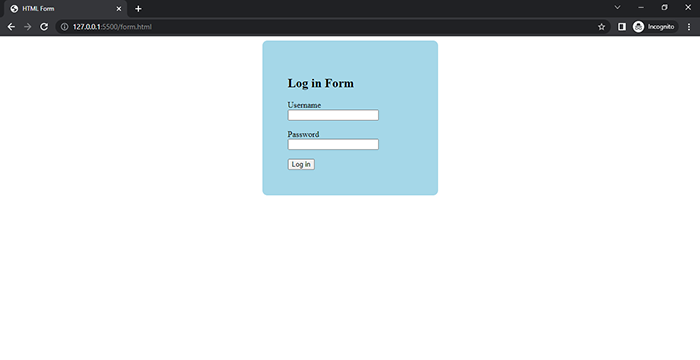
Method 3: Use CSS Position Property
You can use the position property to center the form in both directions horizontally and vertically using CSS. Let’s see how to do it.
In the below CSS code, we set the position property to absolute for the form element. And then we use the left and top properties to center the HTML form.
form{ width: 18%; padding: 50px; border-radius: 10px; background-color: #A5D7E8; position: absolute; left: 37%; top: 20%; }
This time we center the HTML form using CSS not just horizontally but vertically too. The output is in the following image.
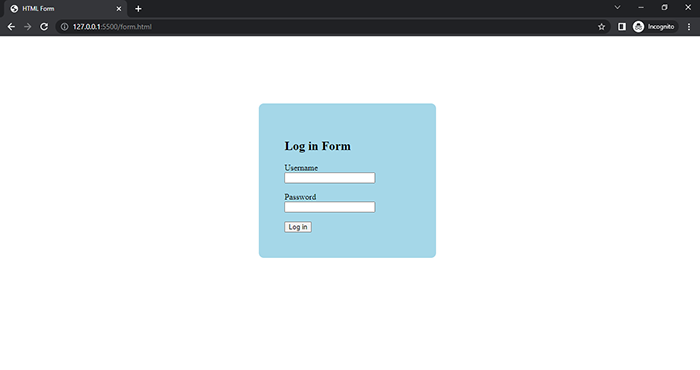
That’s it. Now, you know all three ways to center a form in CSS. If you have any questions related to this tutorial, then just leave a comment below.
And also read:
Don’t forget to help your friends by sharing this tutorial: